Table of Contents
How do I free up Java memory?
Java uses managed memory, so the only way you can allocate memory is by using the new operator, and the only way you can deallocate memory is by relying on the garbage collector.
Does Java automatically free memory?
Java Garbage Collection is the process by which Java programs perform automatic memory management. Java programs compile into bytecode that can be run on a Java Virtual Machine (JVM). When Java programs run on the JVM, objects are created on the heap, which is a portion of memory dedicated to the program.

How do you remove unused objects from memory in Java?
There is no direct and immediate way to free memory in java. You might try to persuade the garbage collector to take away some object using the well known: Object obj = new Object(); // use obj obj = null; System. gc();
How do I fix memory problems in Java?
2) Manually disable & enable parts of your code and observe memory usage of your JVM using a JVM tool like VisualVM.
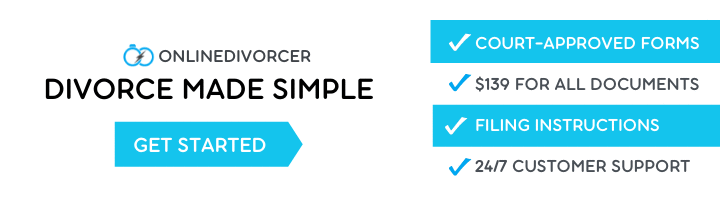
- Make sure that you run it as your own user and not sudo.
- Perform a full update of your system (sudo yum update).
- Reboot helps 🙂
- Try to close all running Java applications.
How do you nullify or remove the unused objects from memory?
In java, garbage means unreferenced objects. Garbage Collection is process of reclaiming the runtime unused memory automatically. In other words, it is a way to destroy the unused objects. To do so, we were using free() function in C language and delete() in C++.
How can you ensure that the memory allocated by an object is freed?
How can you ensure that the memory allocated by an object is freed? By setting all references to the object to new values (say null). Garbage collection cannot be forced. The programmer cannot force the JVM to free the memory used by an object.
How do you empty an object in Java?
You can delete an object in Java by removing the reference to it by assigning null. After that, it will be automatically deleted by the Garbage Collector.
What is Java memory leak?
In general, a Java memory leak happens when an application unintentionally (due to logical errors in code) holds on to object references that are no longer required. These unintentional object references prevent the built-in Java garbage collection mechanism from freeing up the memory consumed by these objects.
Which operator is used to free the memory of an unused object?
delete
5. Operator used to free the memory when memory is allocated? Explanation: ‘New’ is used to allocate memory in the constructors. Hence, we should use ‘delete’ to free that memory.
What is null object in Java?
In object-oriented computer programming, a null object is an object with no referenced value or with defined neutral (“null”) behavior. The null object design pattern describes the uses of such objects and their behavior (or lack thereof).
Does Java have memory leak?
Why do we have to nullify references to variables in Java?
Doing so prevents the Java garbage collector from reclaiming those objects, and results in increasing amounts of memory being used. Explicitly nullifying references to variables after their use allows the garbage collector to reclaim memory.
What happens if you null a list in Java?
Share Follow answered Aug 31 ’12 at 21:11 GothriGothri 2911 bronze badge 1 1 This is actually not true. The Java garbage collector is smart enough to handle that correctly. If you null the List (and the objects within the List don’t have other references to them) the GC can reclaim all the objects within the List.
Does the JVM make your app run slower?
If the JVM pays attention to the “suggestion” to run the GC, it will almost certainly make your app run slower, possibly by many orders of magnitude! – Stephen C Oct 14 ’09 at 22:57 | Show 6more comments 11 *”I personally rely on nulling variables as a placeholder for future proper deletion.