Table of Contents
How do I capture video with OpenCV?
Steps to capture a video:
- Use cv2. VideoCapture( ) to get a video capture object for the camera.
- Set up an infinite while loop and use the read() method to read the frames using the above created object.
- Use cv2. imshow() method to show the frames in the video.
- Breaks the loop when the user clicks a specific key.
How do you make an OpenCV video in Python?
To write a video we need to create a VideoWriter object.
- First, specify the output file name with its format (eg: output. avi).
- Then, we should specify the FourCC code and the number of frames per second (FPS).
- Lastly, the frame size should be passed.
How do I record video on cv2?

The cv2. imshow() method displays a video or image in a window. The window automatically fits the Video or image size. Until we press the q key or 1 key, it will keep opening a web camera and capture the Video.
What is CV VideoCapture?
OpenCV VideoCapture OpenCV provides the VideoCature() function which is used to work with the Camera. We can do the following task: Read video, display video, and save video. Capture from the camera and display it.
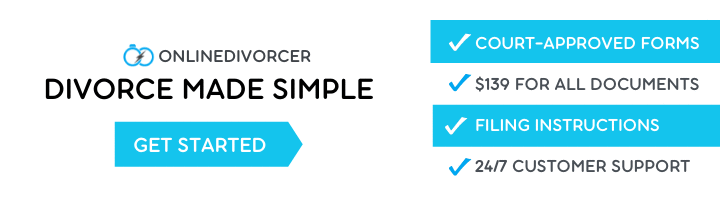
How do I make a video in Python?
“python create video from images” Code Answer
- import cv2.
- import numpy as np.
- # choose codec according to format needed.
- fourcc = cv2. VideoWriter_fourcc(*’mp4v’)
- video = cv2. VideoWriter(‘video.avi’, fourcc, 1, (width, height))
-
- for j in range(0,5):
How do I record a webcam in Python?
How to use:
- Run the file webcam-capture-v1.01.py by running the command python3 webcam-capture-v1.01.py.
- The webcam will start running.
- Bring the picture that you want to save in the webcam frame.
- Once the object is in the right frame, press the key ‘s’ to save a picture.
- If you want to quit, just press ‘q’.
Can we play video in Python?
In order to play youtube videos in python we need pafy and vlc module.
How do I convert frames to video in OpenCV?
Creating Video from Images using OpenCV-Python
- Fetch all the image file names using glob.
- Read all the images using cv2. imread()
- Store all the images into a list.
- Create a VideoWriter object using cv2. VideoWriter()
- Save the images to video file using cv2. VideoWriter().
- Release the VideoWriter and destroy all windows.
How do you make an animated video in Python?
- Import matplotlib modules.
- Import moviepy modules.
- Create a numpy array.
- Create a subplot using matplotlib.
- Create a Video clip file by calling the make_frame method.
- Inside the the make frame method.
- Clear the plot and create a new plot using trigonometry methods according to the frame time.
How do I save an OpenCV file as an MP4?
- import numpy as np.
- import cv2.
- cap = cv2. VideoCapture(0)
- # Define the codec and create VideoWriter object.
- #fourcc = cv2.cv.CV_FOURCC(*’DIVX’)
- #out = cv2.VideoWriter(‘output.avi’,fourcc, 20.0, (640,480))
- out = cv2. VideoWriter(‘output.avi’, -1, 20.0, (640,480))
How do I stream a webcam in Python?
“python get webcam stream” Code Answer
- import cv2.
- cap = cv2. VideoCapture()
- # The device number might be 0 or 1 depending on the device and the webcam.
- cap. open(0, cv2. CAP_DSHOW)
- while(True):
- ret, frame = cap. read()
- cv2. imshow(‘frame’, frame)
- if cv2. waitKey(1) & 0xFF == ord(‘q’):
How to display a video file in Python using OpenCV?
– Learn to read video, display video, and save video. – Learn to capture video from a camera and display it. – You will learn these functions : cv.VideoCapture (), cv.VideoWriter ()
How to access cameras using OpenCV with Python?
– Use cv2.VideoCapture () to get a video capture object for the camera. – Set up an infinite while loop and use the read () method to read the frames using the above created object. – Use cv2.imshow () method to show the frames in the video. – Breaks the loop when the user clicks a specific key.
How to read, write, display videos using OpenCV?
Writing Videos. Let’s now take a look at how to write videos.
How to perform Camera calibration using OpenCV?
opencv-single-camera-calibration. Single camera calibration using opencv library – Python code. This repository contains a simple python code for geometric calibration of single camera. Camera calibration is the process of estimating the transformation matrices from the world frame to the image plane. The calibration is performed by oserving a plane object with particular pattern with known dimensions.